In this article, I will demonstrate the process of dynamically generating unique slugs for any models within Laravel 10. Our approach involves crafting distinctive slugs for tables existing within our Laravel application. By crafting a unified helper method and strategically invoking it, we can seamlessly generate these unique slugs. By establishing a solitary helper method and employing it within the store method of a controller, we gain the ability to effortlessly produce distinct slugs for diverse tables. When utilizing the helper method, it becomes necessary to provide certain parameters to facilitate the dynamic creation of slugs for various models. Let’s follow the steps,
Step 1: Create a helper file
At first, we will create a directory named Helpers inside the app directory. Then create a php file and name it helper.php
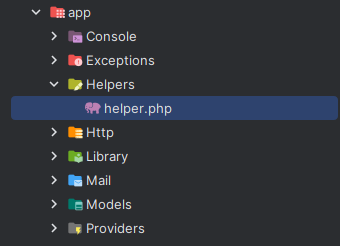
Step 2: Include this helper.php file in the composer.json
Now add the helper.php file in the composer.json file. Open the composer.json file located in the root directory of the laravel project. Then scroll down to the “autoload” section and add the helper.php with its path in the files array. Adding this file, you can access any function written inside this helper.php file across the entire application.
"autoload": {
"psr-4": {
"App\\": "app/",
"Database\\Factories\\": "database/factories/",
"Database\\Seeders\\": "database/seeders/"
},
"files": [
"app/Helpers/helper.php"
]
},
JSONAfter that, run this command.
composer dump-autoload
Step 3: Write the slug-generating function inside the helper file
Let’s enhance the helper function with the following code. In this section, we will incorporate the necessary logic for generating exclusive slugs that are applicable to any given model. Inside the helper.php file,
function create_slug($sluggable_text, $model_name, $column_name = 'slug', $is_module = false, $module_name = null)
{
// Use CamelCase for Model and Module Name
if ($is_module) {
$model_path = 'Modules\\' . ucwords($module_name) . '\Entities\\' . ucwords($model_name);
} else {
$model_path = '\App\Models\\' . ucwords($model_name);
}
$slug = Str::slug($sluggable_text);
$check = true;
do {
$old_category = (new $model_path)->where($column_name, $slug)->orderBy('id', 'DESC')->first();
if ($old_category != null) {
$old_category_name = $old_category->$column_name;
$exploded = explode('-', $old_category_name);
if (array_key_exists(1, $exploded)) {
$number = end($exploded);
if (is_numeric($number) == true) {
$number = (int)$number;
array_pop($exploded);
$final_array = array_merge($exploded, Arr::wrap(++$number));
$slug = implode('-', $final_array);
} else {
$slug .= '-1';
}
} else {
$slug .= '-1';
}
} else {
$check = false;
}
} while ($check);
return $slug;
}
PHPStep 4: Create a unique slug from the controller
During this stage, we will generate distinct slugs for both posts and products by invoking the designated helper method. This versatile helper method can be accessed from any controller, enabling the generation of unparalleled slugs for various tables within the application. Let’s see an example,
PostController.php
Here, we make use of the create_slug()
function from the helper, passing in the text that needs to be converted into a slug, the name of the model, and the designated column name within the Post::create([ ])
method. This operation culminates in the creation of a distinctive slug specifically tailored for the “posts” table.
public function store_post(Request $request)
{
$request->validate([
'name'=>'required|max:191',
'slug'=>'required|max:191',
]);
// If the column name is slug
Post::create([
'name' => $request->name,
'slug' => create_slug($request->slug, 'Post'),
]);
// If the column name is different, Do this
Post::create([
'name' => $request->name,
'your_column_name' => create_slug($request->slug, 'Post', 'your_column_name'),
]);
}
PHPOptional Step: Create a unique slug inside a module from the controller
If you wish to employ this function within a Laravel module, you can seamlessly integrate it. Let’s consider a hypothetical scenario where you have a module named “ProductModule” having a model named “Product.” In this case, the model corresponds to the “products” table, with the designated column being “slug.”
// Inside the controller of ProductModule Module
public function store_product(Request $request)
{
$request->validate([
'name'=>'required|max:191',
'slug'=>'required|max:191',
]);
Product::create([
'name' => $request->name,
'slug' => create_slug($request->slug, 'Product', 'slug', true, 'ProductModule'),
]);
// Your Extra Logic
}
PHPBy using this method, you can generate unique slugs for your entire application, which can speed up your development process.